It’s been a while since I worked with WordPress, especially building themes. This time around, I wanted to bring in a bit more modern development experience into the process. I wanted to build a Single Page Application (SPA), with WordPress’ Rest API – but as a WordPress theme. The problem is, this can be a pain to setup. Especially with the build step and all.
I specifically wanted to use React for the front end. React is Facebook’s product, and per their website: React is a library for building user interfaces. It has a very broad user base and lots of modules available, which makes it ideal for our theme. In conjunction, we’re using create-react-wptheme – which will make our theme up and running with React in no time. And of course, WP Rest API for the backend.
Note that this tutorial is geared towards PHP or WordPress developers – who are looking to get started working with Single Page Applications with React.
This will be the first of a series of posts:
The theme we’re going to build throughout this series is more of a starter theme. We’re calling it “barebones” and it contains just the right amount of functionality for a basic WordPress theme.
We will need the following to get started:
- nodejs + npm
- git bash (or terminal)
- local WordPress installation
create-react-wptheme
Let’s talk briefly about create-react-wptheme. The goal is to get us bootstrapped with a new React based WordPress theme with a few commands. If any of you are familiar with create-react-app, its basically the same functionality – but for WordPress. One primary difference is that it uses WordPress (not webpack), as the development server. This makes development consolidated in one – front end and back end.
In addition, since it’s a WordPress theme, you have access to all the core functions, filters, actions, hooks etc. Also, you can use WordPress’ nonce for authenticated requests. Lastly, if you must use plain PHP – say only for a specific page, you can still use WordPress’ page templates – which is very handy.
So with that in mind, let’s get started.
First, assuming you have a local WordPress installation, go ahead and start a terminal (git bash) in the themes directory.
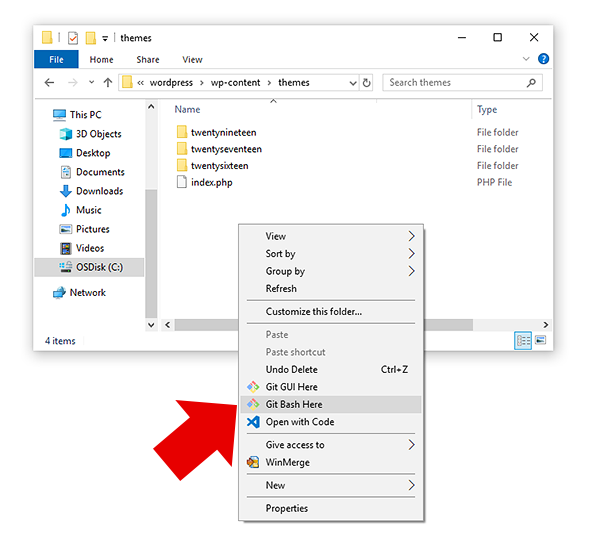
In Windows, git bash is a pretty good tool, simply right click and “Git Bash Here”. This will launch the terminal, where we can start our installation. Type in the command below:
npx create-react-wptheme barebones
Note that “barebones” is the name of our theme. You can simply replace this with a theme name of your preference.
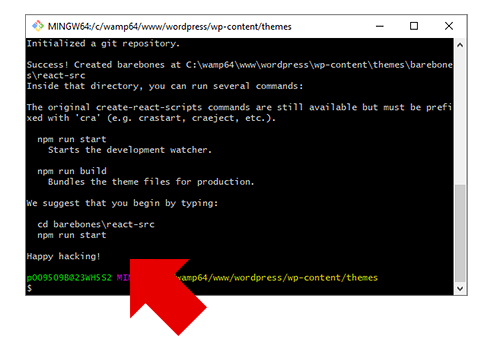
Once that’s done, you will see a message like above. The installation created a root folder, with a “react-src” directory inside it. Consider the react-src directory as the most important directory because it holds all of your un-compiled code. From this directory – we can build the rest. You’ll see what I mean later.
Note that at this step, our theme is not ready yet.
See, if you look inside wp-admin > themes, you will see “barebones” under the “Broken Themes” section.
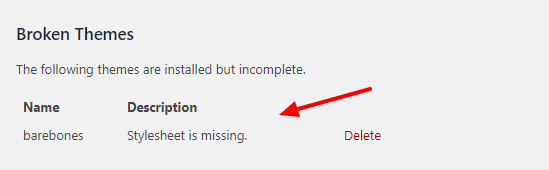
This is because we don’t have the necessary files (mainly the styles.css) for it to be a valid theme. Note that we also need index.php, so the we can hold the JavaScript and CSS files together.
wpstart
Let’s go back to our terminal and type the following:
cd barebones/react-src
npm run wpstart
We’re going into our theme directory and inside “react-src” by using the “cd” command, then we run wpstart. This will fix the “Broken Themes” issue, and if we go back to the browser and go in wp-admin > themes themes, you should be able to see our theme.
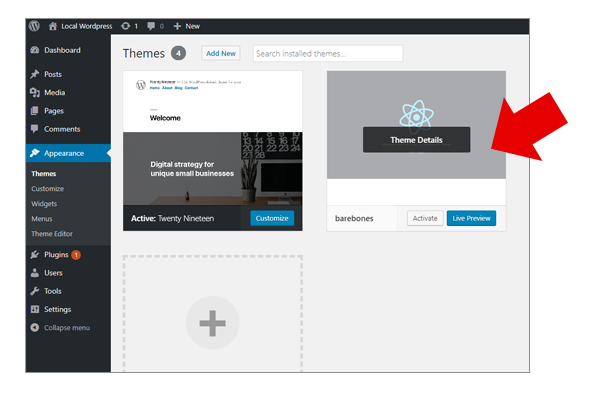
Now let’s activate the theme by clicking “Activate“. This will tell WordPress to use this theme we just built. Now let’s go and view our site in the browser. You should see a message
“Please restart the Nodejs watcher now… “
What this means is that we have to run wpstart a second time, for the script finish setting things up. Let’s go back to git bash and do a “CTRL + C”. Then type in:
npm run wpstart
Now, once this is done, a new browser tab should have opened automatically and looks like below:
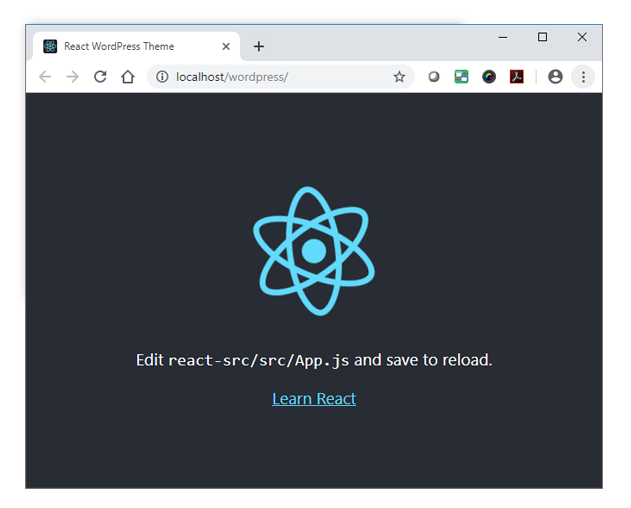
It may not look like much, but this tells us a lot. This page indicates that we’ve just successfully installed our React theme. This includes the PHP, CSS and JavaScript files, plus all the resources to run our React application. So well done!
Note: wpstart is for “dev” mode.
From this point onward, when you’re in wpstart mode, (when you do npm run wpstart) that means you are in development mode. What this means is that anytime you change something in the react-src directory, the files will get recompiled and placed in the proper places. Any changes will also cause your browser to refresh – so you see your changes instantly.
Do Not Edit the files in ROOT
The files in the root folder (outside of react-src), is the compiled version of your code that is needed for WordPress and React to run. You shouldn’t edit anything in here because as soon as you save files in react-src – the files in the root will be replaced with the new. So anything you change here will get OVERWRITTEN.
File Structure
Let’s take a quick look at at the file structure for it’s important to know what it is and how create-react-wptheme use it. In a regular WordPress theme, all we really need are the PHP (such as header, footer) and CSS.
In our new theme, it looks something like below:
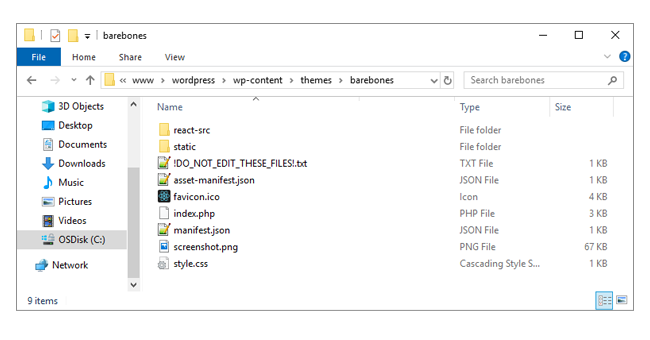
As you can see, there is none of the familiar files you would expect in a WP theme. Remember we’re building an SPA – which will all be in JavaScript.
As mentioned previously, inside react-src are the uncompiled and “editable” version of your code. Everything else (the root and static folder) are the output of what you have in react-src. Take note of that text file that’s titled !DO_NOT_EDIT_THESE_FILES!.txt. Remember what I said about not editing files in the root?
The “public” folder
The author of create-react-wptheme saved a special folder for our non-react files called “public”. Whatever you add in this folder, gets copied directly to the root. So, things like functions.php, or page templates – even CSS or JS can be dumped in here – and it will get copied into the root at compile time.
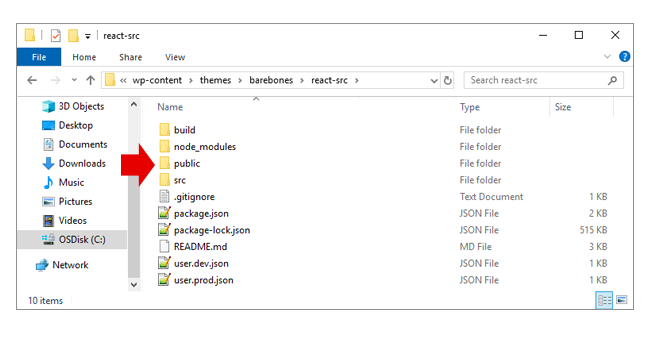
Again, this folder can be extremely helpful – especially for developers who would still like to access core functionality such as hooks, filters, actions etc.
Also, index.php – will only get loaded once, and is the entry way for your React application. The goal is, once loaded, all interactions will be through the REST api. So whatever PHP has produced in index.php will stay the same all throughout your application (except PHP page templates).
The “build” folder
We haven’t covered wpbuild yet, but since we talking about the file structure, you will notice a folder called “build“. This is a special folder that holds the final “deployable” code.
What that means is, almost like the contents of the “root” folder, but compressed, minified and optimized for production. Also, it doesn’t contain any dev files (such as react-src).
This brings us to the last section: wpbuild:
wpbuild
So let’s get back to git bash and do CTRL + C. Type in the following command:
npm run wpbuild
You will see messaging that looks something like:
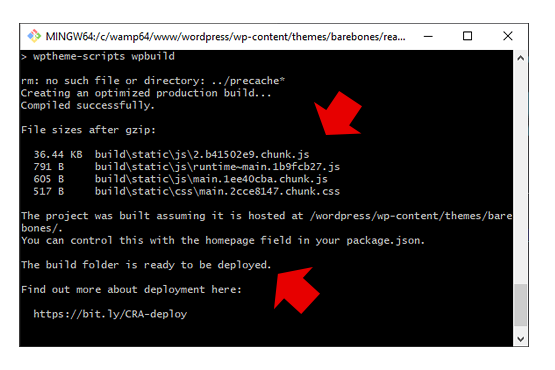
This simply shows files that have been created, optimized and placed in the build folder, as well as the root. This means that you see your optimized code right away.
To see how that looks, when you refresh your browser, you will see just a bunch of compressed code like this:
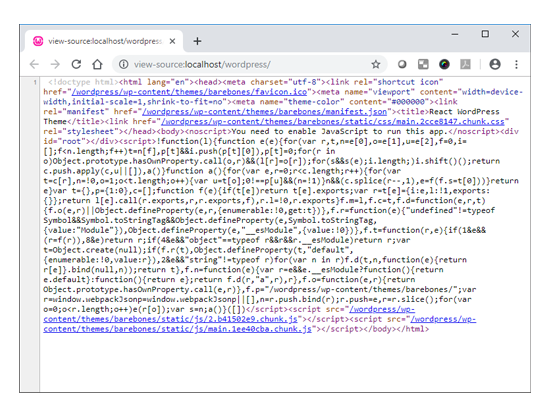
Your WordPress theme’s source code has been flattened, ready for world consumption. Also, take a look at the contents of the root directory:
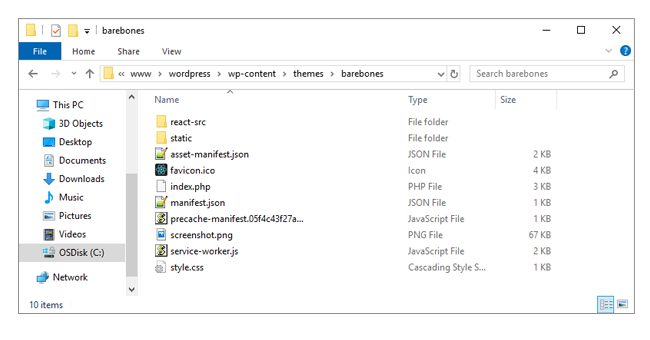
You notice the absence of the file !DO_NOT_EDIT_THESE_FILES!.txt. That means, that you’ve just run wpbuild and its now in “build” mode.
When you’re ready to go back to making some changes, don’t forget to go back into “dev” mode, by running “npm run wpstart” in the react-src directory.
And there you have it. We have our React application running as a WordPress theme. I’ve created a Github repo for Barebones theme. You can go ahead and fork it for your next project, or stay tuned for more tutorials.
Thank you for the article and for sharing knowledge!. I’ve been wondering how to use ReactJS for developing WordPress theme and these series come in handy.
Really really thanx for the article, i have been looking for any tutorials about wp and ReaxtJS but not even one explains like this, again thanx for sharing your knowledge!👍👍
It doesn’t work fully on my part, the problem is that after running build it doesn’t generate files in a root of a theme but inside another subfolder with a same name? I have tried it two times hm.. https://i.imgur.com/VDbo2OT.png
It works after manually moving generated files to a root directory. I am on Windows if that matters?
Sorry for the confusion. This article is meant for create-react-wptheme – which is what “Barebones” is built with. To use Barebones -> use the instruction on this page: https://github.com/michaelsoriano/barebones
Hey guys i appreciate this article. Maybe anyone can help me. I dont’ really no where should i place the css files in order to have them after the build precess. I alway get the ‘Stylesheet is missing.’ error.
Thanks 🙂
The CSS files can be located anywhere in your react-src directory. This is up to you to structure. The “Stylesheet” missing error – is because it hasn’t done the “build process yet”.
Do:
cd barebones/react-src
npm run wpstart
When I visit https://myblog.wordpress.com/wp-admin/themes.php after running npm run wpstart I cannot see the barebones theme there. Do I need to install a PHP server as well as installing wordpress locally? Thank you for your post!
Yes – this tutorial is for local WP installation.
Great. How does it works if you deploy on goDaddy ? I already using WordPress but I would like to redo it entirely in react as I think it will be better than adding plugin on top of plugin and on and on
Hi, I try to install react-wptheme in remote server but I receive this error and installation fails:
The directory react-src contains files that could conflict:
package.json
Either try using a new directory name, or remove the files listed above.
Can You help me? I would try this road for wp theme dev. Thank you in advance for anyone who respond me
Sounds like you are installing React in a directory that is already a javascript project.
Make sure to “cd” into a new unique directory to install a new react app.
Would this work with WPGraphQL?
Hi did you try using this with WPGraphQL? Did it work for you?
No I haven’t
after generating the files for the theme. I upload it to my site using ftp and then I can activate the site and then it doesn’t work. The interesting thing is that it works locally tho.
i’m having the same trouble when uploading the build folder with ftp, anyone knows how to make it work in live wordpress? Thanks!
did you find solution ?
HI,
i have a problem with minification. When I deploy my files, everything works fine but when I use developer tools in my chrome browser i can see that my files aren’t minified. All class and functions names are as they where before building app. How can I fix this – how to minify theese files?
did you try npm run wpbuild?
Hello.! can deploy this react theme without not “npm run wpbuild” command .. because when i deploy this project using this command .. i can’t change anything in this code .. so can i deploy development folder structure ?
please help me … my project is done
i want to deploy wordpress project with react theme on shared hosting without run npm run wpbuild command ..anyone here which do this soo please help me … thanks in advance
Hi! Great post! Thanks… After run this command npm run wpbuild I have a message Stylesheet is missing. and when i go back to dev mode everything is fine. How can I fix this?
Thanks for this amazing post Michael. Actually I created a theme with npm react-create-wptheme as mentioned above and also I have completed the development part of the theme. I am facing issue in pages when I refresh the pages except Home page it shows 404 error. I am using React Router V6.
A help from you would be really appreciated mate 🙌🏻
Thanks
You should use HashRouter instead of BrowserRouter. WordPress has it’s own router, so if you user regular browser router it will break