From time to time, I write real generic articles about JavaScript that may be common knowledge and/or that is featured in other websites and videos. These articles really serve myself a purpose. More like a “note to myself” – so instead of searching, I just go to my website and voila!
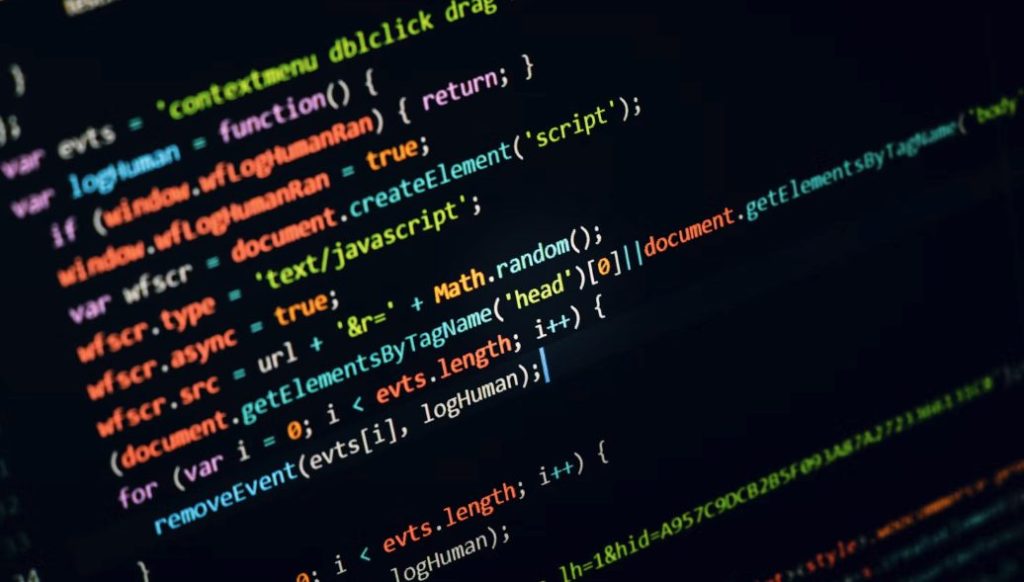
Today it’s one of those articles. We’re going to look at a few super useful JavaScript features that can make your coding life (and mine) a whole lot easier. Whether you’re just starting out or you’ve been coding for a while, these tools are worth adding to your toolkit.
Let’s dive in!
1. Object.hasOwn(): A Safer Way to Check for Properties
Have you ever needed to check if an object has a specific property? Maybe you’ve used the in
operator or Object.prototype.hasOwnProperty()
before. Well, Object.hasOwn()
is here to make that task simpler and safer.
How it works:
Object.hasOwn(object, propertyName)
You pass in the object you want to check and the name of the property you’re looking for. It returns true
if the object has that property as its own (not inherited), and false
otherwise.
Why it’s cool:
Unlike Object.prototype.hasOwnProperty()
, Object.hasOwn()
works even if the object doesn’t inherit from Object.prototype
or if it has its own hasOwnProperty
method. It’s like a superhero version of hasOwnProperty
!
Before Object.hasOwn():
const person = { name: "Alice" };
// Old way (potentially unsafe)
if (person.hasOwnProperty("name")) {
console.log("Person has a name");
}
// Safer old way
if (Object.prototype.hasOwnProperty.call(person, "name")) {
console.log("Person has a name");
}
With Object.hasOwn():
const person = { name: "Alice" };
if (Object.hasOwn(person, "name")) {
console.log("Person has a name");
}
Much cleaner, right?
2. String.replaceAll(): Find and Replace Made Easy
This one is a game changer. I’m sure you’ve run into the old school replace – where it only does the first occurrence. I’m sure you’ll always almost need to replace ALL occurrences right? No more messing with regular expressions or some clever looping. Now, it’s as easy as pie!
How it works:
string.replaceAll(searchValue, replaceValue)
You provide the substring you want to replace and what you want to replace it with. It returns a new string with all matches replaced.
Why it’s awesome:
It’s straightforward and doesn’t require you to mess with regular expressions for simple replacements. Plus, it replaces ALL occurrences, not just the first one like replace()
does.
Before String.replaceAll():
const sentence = "The quick brown fox jumps over the lazy dog. The fox is quick!";
// Using regular expression
const newSentence = sentence.replace(/fox/g, "cat");
// Or using split and join
const newSentence2 = sentence.split("fox").join("cat");
With String.replaceAll():
const sentence = "The quick brown fox jumps over the lazy dog. The fox is quick!";
const newSentence = sentence.replaceAll("fox", "cat");
So much easier to read and understand!
3. structuredClone(): Deep Cloning Made Simple
Another good one that will blow your mind. Let’s talk about structuredClone()
. If you’ve ever tried to create a deep copy of an object in JavaScript, you know it can be tricky. This function makes it a breeze!
How it works:
const clone = structuredClone(original)
You pass in the object you want to clone, and it returns a deep copy of that object.
Why it’s fantastic:
It can handle circular references, and it works with a wide variety of built-in types (like Date, Map, Set, and more). It’s also typically faster than other deep cloning methods.
Before structuredClone():
Can you say “yuck”?
const original = {
name: "Alice",
pets: ["dog", "cat"],
details: { age: 30 }
};
// Using JSON (loses some data types)
const jsonClone = JSON.parse(JSON.stringify(original));
// Or a custom recursive function (complex and error-prone)
function deepClone(obj) {
if (typeof obj !== "object" || obj === null) return obj;
const newObject = Array.isArray(obj) ? [] : {};
for (let key in obj) {
newObject[key] = deepClone(obj[key]);
}
return newObject;
}
const customClone = deepClone(original);
With structuredClone():
const original = {
name: "Alice",
pets: ["dog", "cat"],
details: { age: 30 }
};
const clone = structuredClone(original);
It’s like magic, isn’t it?
4. Array.at(): Flexible Array Index Access
Last one: ever wanted to access array elements from the end without knowing the array’s length? I’m sure you’ve done this hundreds of times. Well with Array.at()
– your development life is going to be a tad better.
How it works:
array.at(index)
You provide an index, which can be positive or negative. Positive indices work like normal array access, while negative indices count from the end of the array.
Why it’s great:
It provides a clean way to access elements relative to the end of an array without needing to know its length. It’s especially useful for accessing the last few elements of an array.
Before Array.at():
const fruits = ['apple', 'banana', 'cherry', 'date'];
// Getting the last element
const lastFruit = fruits[fruits.length - 1];
// Getting the second to last element
const secondToLastFruit = fruits[fruits.length - 2];
With Array.at():
const fruits = ['apple', 'banana', 'cherry', 'date'];
// Getting the last element
const lastFruit = fruits.at(-1);
// Getting the second to last element
const secondToLastFruit = fruits.at(-2);
It’s more readable and less error-prone, especially when dealing with array lengths!
And there you have it! Super handy JavaScript features that can make your code cleaner, safer, and more efficient. Give them a try in your next project and see how they can level up your coding game.
Before you go, you might also find this one useful: Common JavaScript snippets for data manipulation
Happy coding!