Still working with Next.js and WordPress here – as described in my previous post. I’m at the single post now, and as it turned out – it’s really easy to build the basic functionality. So in Next.js – you simply add a component in the “Pages” directory to “route” the requests. For dynamic routes – simply enclose the page name inside brackets – and this is what you name the dynamic parameter.
In our case, the “slug“. So our permalink would look something like below:
/post/slug-of-the-post
I’ve created a folder called “post” – which matches the first section of our permalink /post/. The second part of the path – is the dynamic part, which Next.js passes into our component to handle.
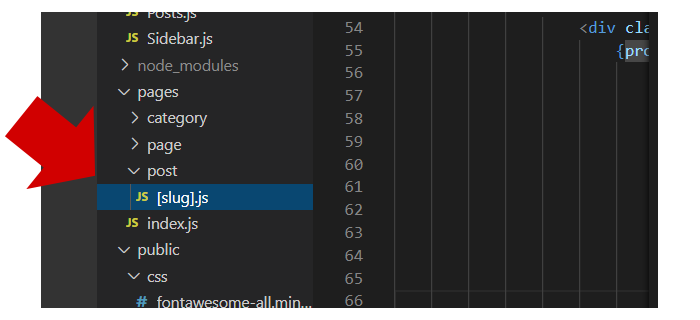
Once that’s in place, the code looks something like below. First, we import the necessary modules:
import Axios from 'axios'
import React, { Fragment } from 'react'
We use Axios for our http calls. And I like to use Fragments – to still enclose our HTML inside a parent element – but it doesn’t output unnecessary DIVs.
Next, let’s create our getServerSideProps() – which sets up the props we can utilize in our component “[slug].js”.
export async function getServerSideProps(context) {
const url = 'http://yourwordpress.url/wp-json/wp/v2/';
const slug = context.query.slug;
const posts = await Axios.get(url + `posts?slug=${ slug }`);
const post = posts.data.length > 0 ? posts.data[0] : null;
let output = {
props: {
post : post
}
}
if(!post){
context.res.statusCode = 404;
return output;
}
return output;
}
Look familiar? Yes – its React – but server side. In the function above, we’re setting up what we’re returning as the “props” – to be used in our default exported function (the component).
export default ({ ...props }) => {
return (
<Fragment>
<div id="wrapper">
<div id="main">
{props.post === null ? (
<h1>Not found</h1>
) : (
<Fragment>
<h1>{props.post.title.rendered}</h1>
<article dangerouslySetInnerHTML={ { __html : props.post.content.rendered } } />
</Fragment>
)}
</div>
</div>
</Fragment>
)
}
The code above is a simple HTML that works with the props we’ve setup. It simply shows the content of our API call (if post is found).
And that is basically the meat of it.
The next parts will be to add more features such as a comment list, and a form.
More to come.