I was given a task to create a simple API that would fetch jobs from our new system Workday. This API would accept a few parameters, and spit out the relevant jobs from it. My thoughts are always – if I was to build something, might as well learn something new in the process.
I’ve always wanted to pickup more AWS technologies, because it seems that that’s where all of development is heading. Everything now is in the cloud. Not just SaaS, but we also have FaaS – which stands for Function as a Service.
So how was it? First, off – in the expense of not having a server, you would need to learn how to get around in AWS UI. If you can find Lambda, you will be presented with a screen to create your first function.
Then there is the designer. Which I’m not sure I like very much. It’s a bit confusing – with the “flowchart” like layout etc. I see that the function is at the very top – and everything else stems from it. Hmmm.
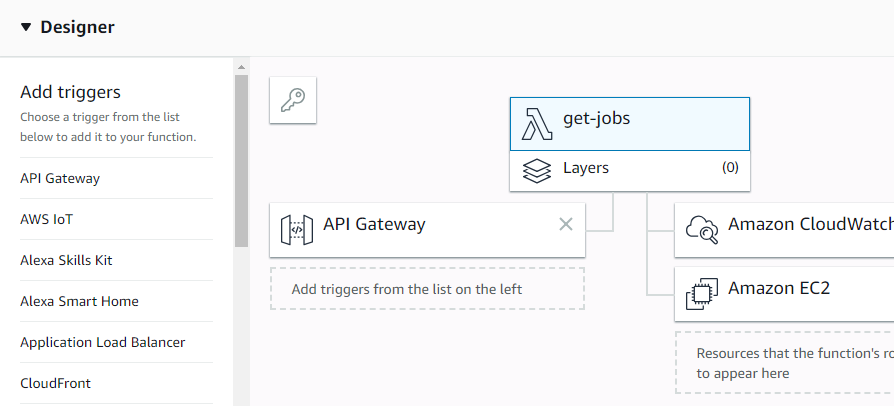
On the other end of the spectrum, we have the API Gateway. This is where the public actually get’s to hit your function.
There is a resources section – where you actually get to see the stages of your call. From configuring the Request, all the way to the response. It has a an interface that outlines the whole process. I’m not sure how I feel about it either.
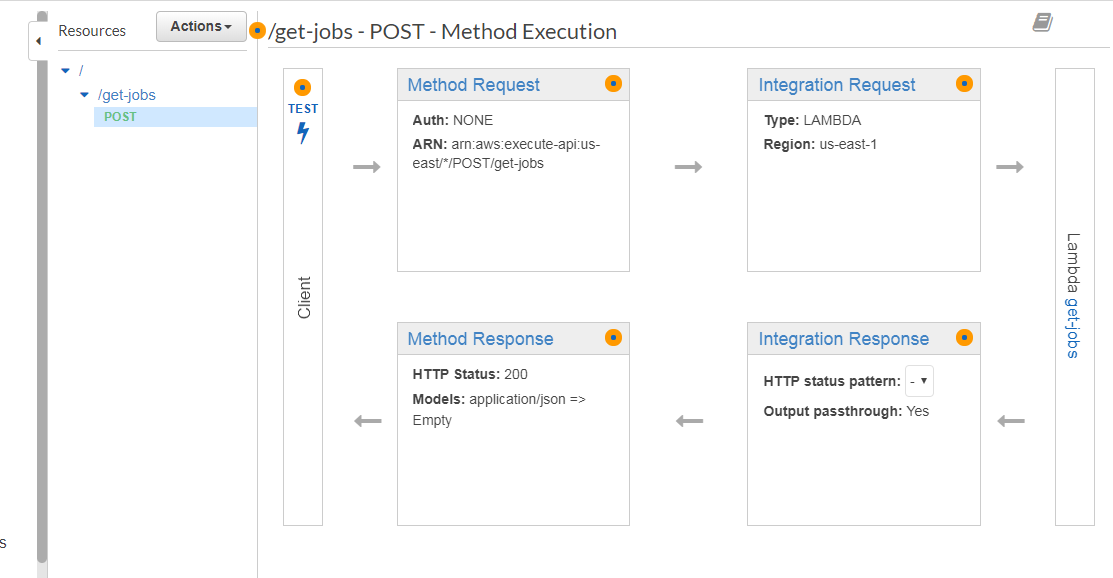
Okay, let’s see how I did. First of all, I used NodeJS to build my small function. All it does is make an “https” call to another API. Accept a few parameters, and filter the response according to it.
The Function
As you can see below, it’s a simple snippet of code. Everything is wrapped around the handler. This basically sets up the https module and executes it. The data that comes back is stuffed into a variable called “data”.
const https = require('https');
exports.handler = (event, context, callback) => {
let options = {
host : 'somesite',
path: '/path1/'
};
const req = https.request(options, (res) => {
let body = '';
// console.log('Status:', res.statusCode);
// console.log('Headers:', JSON.stringify(res.headers));
res.setEncoding('utf8');
res.on('data', (chunk) => body += chunk);
res.on('end', () => {
console.log('Successfully processed HTTPS response');
});
});
req.on('error', callback);
req.write(JSON.stringify(event));
req.end();
};
Quick note: the Cloud9 editor – is one of the best I’ve seen. I am really impressed with it. It’s very solid, has plenty of features and parallels many of the desktop IDE’s / Text editors that I’ve used.
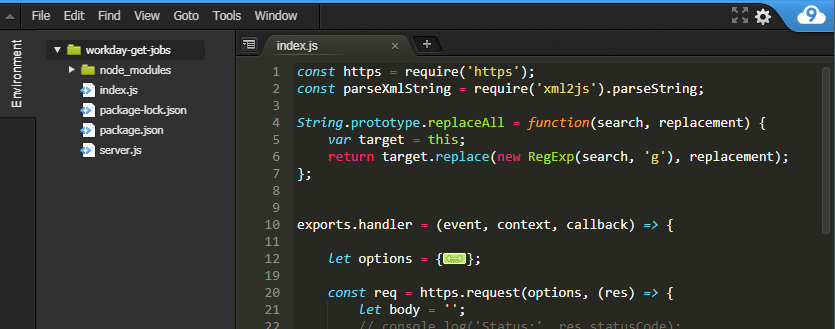
The parameters that is passed into the function is in the object called “event”. Which I’m not really going into at the moment. This is basically a general overview of my function, and the steps to make it work.
Although Cloud9 is good, the Labmda UI works, it still doesn’t replace working locally. The steps that you have to go through – testing your code, changing params, clicking the buttons here and there – it’s still just too slow.
Working Locally
So I had to get this same project locally. Lambda has an option of uploading a .zip file, so that’s what I did.
I also have a server.js file – which is not part of the API – but a file that I call locally to make the whole thing work in my machine.
Basically, what this is doing is running a server locally, and calling our Lambda function directly. Notice the line “index.handler…”
var http = require('http');
var index = require('./index');
var { parse } = require('querystring');
process.env["NODE_TLS_REJECT_UNAUTHORIZED"] = 0;
var httpserver = http.createServer(function(req,res){
let params = '';
req.on('data', chunk => {
params += chunk.toString(); // convert Buffer to string
});
req.on('end', () => {
if(req.headers['content-type'] == 'application/x-www-form-urlencoded') {
index.handler(parse(params),"",function(empty, result){
res.setHeader('Content-Type', 'application/json');
res.write(JSON.stringify(result));
res.end("");
});
}else{
res.end("No params passed");
}
});
});
var port = 5050;
httpserver.listen(port,function(){
console.log('Server is running on port -> ' + port);
});
So now, I can run my function without having to go through the Lambda API UI to test, reload etc.
All I need is to run
nodemon server.js
Nodemon is a NodeJS module that automatically restarts and reloads your server when you save.
Also, I use PostMan, and save both endpoints (local and live), to track the results etc.